mkdir profile
rem --- create ls command ---
echo @echo off > profile/ls.bat
echo dir >> profile/ls.bat
rem --- create pwd command ---
echo @echo off > profile/pwd.bat
echo cd >> profile/pwd.bat
Tuesday, November 11, 2008
Windows CMD prompt profile for Linux users
rem Set PATH to current working directory
Wednesday, October 22, 2008
Makefile Tips and Tricks - GNU Make
http://www.gnu.org/software/make/manual/make.html
Index of Concepts
append '-' before command to ignore error in makefile and
--silent
with make or '@' before every command to echo off eg:
 
-rm
foo 
-include
Makefile_vars inc.mk  
@echo
" Only once printed ";Implicit variables
MAKELEVEL - Gives level of the make recursive subdir entries
Appendix A Quick Reference
Function Call Syntax
A function call resembles a variable reference.
It looks like this:
$(function arguments)
or like this:
${function arguments}
Functions for Transforming Text
1.Test Functions for String Substitution and Analysis
$(subst
from,to,text
)$(patsubst
pattern,replacement,text
)$(strip
string
).........and so on
example : override CFLAGS += $(patsubst %,-I%,$(subst :, ,$(VPATH)))
2. So on.. Please click link for further details
.PHONY Target
Automatic Variables
$< $@ $? $*
Defining and Redefining Pattern Rules
Here are some examples of pattern rules actually predefined in make. First, the rule that compiles `.c' files into `.o' files:
%.o : %.c
        $(CC) -c $(CFLAGS) $(CPPFLAGS) $< -o $@
defines a rule that can make any file x.o from x.c. The command uses the automatic variables `$@' and `$<' to substitute the names of the target file and the source file in each case where the rule applies (see Automatic Variables).
Bash Script Tips
http://www.hsrl.rutgers.edu/ug/shell_help.html
Command line arguments to shell scripts are positional variables:
$0, $1, ... - The command and arguments.
   With $0 the command and the rest the arguments.
$# - The number of arguments.
$*, $@ - All the arguments as a blank separated string.
  Watch out for "$*" vs. "$@".
  And, some commands: shift
$$ - Current process id.
$? - The exit status of the last command. and return result of last function
Conditional Reference
${variable-word} - If the variable has been set, use it's value, else use word.
POSTSCRIPT=first;
echo POSTSCRIPT
POSTSCRIPT=${POSTSCRIPT-second};
export POSTSCRIPT
echo POSTSCRIPT
${variable:-word} - If the variable has been set and is not null, use it's value, else use word.
These are useful constructions for honoring the user environment.
Ie. the user of the script can override variable assignments. Cf. programs like lpr(1) honor the PRINTER environment variable, you can do the same trick with your shell scripts.
${variable:?word} -If variable is set use it's value, else print out word and exit. Useful for bailing out.
String concatenation
The braces are required for concatenation constructs.
$p_01 - The value of the variable "p_01".
${p}_01 - The value of the variable "p" with "_01" pasted onto the end.
Include configuration
. command
This runs the shell script from within the current shell script. For example:
# Read in configuration information
. /etc/hostconfig
Debugging
The shell has a number of flags that make debugging easier:
sh -n command -
Read the shell script but don't execute the commands. IE. check syntax.
sh -x command
Display commands and arguments as they're executed. In a lot of my shell scripts you'll see
# Uncomment the next line for testing
# set -x
Command line arguments to shell scripts are positional variables:
$0, $1, ... - The command and arguments.
   With $0 the command and the rest the arguments.
$# - The number of arguments.
$*, $@ - All the arguments as a blank separated string.
  Watch out for "$*" vs. "$@".
  And, some commands: shift
$$ - Current process id.
$? - The exit status of the last command. and return result of last function
Conditional Reference
${variable-word} - If the variable has been set, use it's value, else use word.
POSTSCRIPT=first;
echo POSTSCRIPT
POSTSCRIPT=${POSTSCRIPT-second};
export POSTSCRIPT
echo POSTSCRIPT
${variable:-word} - If the variable has been set and is not null, use it's value, else use word.
These are useful constructions for honoring the user environment.
Ie. the user of the script can override variable assignments. Cf. programs like lpr(1) honor the PRINTER environment variable, you can do the same trick with your shell scripts.
${variable:?word} -If variable is set use it's value, else print out word and exit. Useful for bailing out.
String concatenation
The braces are required for concatenation constructs.
$p_01 - The value of the variable "p_01".
${p}_01 - The value of the variable "p" with "_01" pasted onto the end.
Include configuration
. command
This runs the shell script from within the current shell script. For example:
# Read in configuration information
. /etc/hostconfig
Debugging
The shell has a number of flags that make debugging easier:
sh -n command -
Read the shell script but don't execute the commands. IE. check syntax.
sh -x command
Display commands and arguments as they're executed. In a lot of my shell scripts you'll see
# Uncomment the next line for testing
# set -x
Makefile Options
http://www.hsrl.rutgers.edu/ug/make_help.html
dependecy1: dependencyA dependencyB ... dependencyN
[tab] command for dependency1
That is probably one of the simplest makefiles that could be made. When you type make, it automatically knows you want to compile the 'myprogram' dependency (because it is the first dependency it found in the makefile). It then looks at mainprog.cc and sees when the last time you changed it, if it has been updated since last you typed 'make' then it will run the 'gcc mainprog.cc ..." line. If not, then it will look at myclass.cc, if it has been edited then it will execute the 'gcc mainprog.cc ..." line, otherwise it will not do anything for this rule.
Before issuing any command in a target rule set there are certain special macros predefined.
1. $@ is the name of the file to be made.
2. $? is the names of the changed dependents.
So, for example, we could use a rule
printenv: printenv.c
[tab] $(CC) $(CFLAGS) $? $(LDFLAGS) -o $@
alternatively:
printenv: printenv.c
[tab] $(CC) $(CFLAGS) $@.c $(LDFLAGS) -o $@
There are two more special macros used in implicit rules. They are:
3. $< the name of the related file that caused the action.
4. $* the prefix shared by target and dependent files.
Example Target Rules
INC=../misc
OTHERS=../misc/lib.a
$(OTHERS):
[tab] cd $(INC); make lib.a
Beware:, the following will not work (but you'd think it should)
INC=../misc
OTHERS=../misc/lib.a
$(OTHERS):
[tab] cd $(INC)
[tab] make lib.a
Each command in the target rule is executed in a separate shell. This makes for some interesting constructs and long continuation lines.
dependecy1: dependencyA dependencyB ... dependencyN
[tab] command for dependency1
That is probably one of the simplest makefiles that could be made. When you type make, it automatically knows you want to compile the 'myprogram' dependency (because it is the first dependency it found in the makefile). It then looks at mainprog.cc and sees when the last time you changed it, if it has been updated since last you typed 'make' then it will run the 'gcc mainprog.cc ..." line. If not, then it will look at myclass.cc, if it has been edited then it will execute the 'gcc mainprog.cc ..." line, otherwise it will not do anything for this rule.
Before issuing any command in a target rule set there are certain special macros predefined.
1. $@ is the name of the file to be made.
2. $? is the names of the changed dependents.
So, for example, we could use a rule
printenv: printenv.c
[tab] $(CC) $(CFLAGS) $? $(LDFLAGS) -o $@
alternatively:
printenv: printenv.c
[tab] $(CC) $(CFLAGS) $@.c $(LDFLAGS) -o $@
There are two more special macros used in implicit rules. They are:
3. $< the name of the related file that caused the action.
4. $* the prefix shared by target and dependent files.
Example Target Rules
INC=../misc
OTHERS=../misc/lib.a
$(OTHERS):
[tab] cd $(INC); make lib.a
Beware:, the following will not work (but you'd think it should)
INC=../misc
OTHERS=../misc/lib.a
$(OTHERS):
[tab] cd $(INC)
[tab] make lib.a
Each command in the target rule is executed in a separate shell. This makes for some interesting constructs and long continuation lines.
Tuesday, October 21, 2008
GCC Options and GDB notes
gcc example.c [options]
-S - Compiles and stops. Output is assembler code (suffix .S).
-o [name] - Gives executable as 'name'.
-E - Preprocess source file only.
    Comments will be discared unless -C is also specified.
    Creates #line directives unless -P is also specified.
-M - The preprocessor outputs one make rule containing the object file name for that source file, a colon, and the names of all the included files,including those coming from -include or -imacros command line options.
-W - Print extra warning messages.
    -Wall print all warnings
Example:
cat > example.c << EOF
#include <
#include <
int main()
{
printf("Hello world");
}
EOF
#
GDB notes:
Compile C code with -g option and run 'gdb a.out'
In gdb prompt set the break point as 'break main' or any function.
To run 'run '
To watch the particular variable set 'watch' and if the variable get modified then it will get notified
To see the local variables 'info local'
To proceed next line 'n or next'
list to display the source code
-S - Compiles and stops. Output is assembler code (suffix .S).
-o [name] - Gives executable as 'name'.
-E - Preprocess source file only.
    Comments will be discared unless -C is also specified.
    Creates #line directives unless -P is also specified.
-M - The preprocessor outputs one make rule containing the object file name for that source file, a colon, and the names of all the included files,including those coming from -include or -imacros command line options.
-W - Print extra warning messages.
    -Wall print all warnings
Example:
cat > example.c << EOF
#include <
stdio.h
>#include <
string.h
>int main()
{
printf("Hello world");
}
EOF
#
GDB notes:
Compile C code with -g option and run 'gdb a.out'
In gdb prompt set the break point as 'break main' or any function.
To run 'run
To watch the particular variable set 'watch
To see the local variables 'info local'
To proceed next line 'n or next'
list to display the source code
Wednesday, July 23, 2008
Intel hex code Format
The Intel hex record looks like:
:090160001204283119740280E236
Intel hex data record: ':nnaaaattddddddd...dcc'
where:
: == start of data record
nn == number of bytes in record in ascii hex
aaaa == address to load data record at in memory
tt == type of record; 00=data; 01=end of file
ddd...d == data bytes of record in ascii hex (two chars per byte)
cc == checksum of record
checksum is two's complement of eight bit sum of all data from 'nn'
to end of data '...d'
end of file record (00) has zero number of bytes
So the above record would be decoded as :
:090160001204283119740280E236
start of record :
length of record 09
address to store record at 0160
record type 00
actual data of record 1204283119740280E2
checksum of record 36
:090160001204283119740280E236
Intel hex data record: ':nnaaaattddddddd...dcc'
where:
: == start of data record
nn == number of bytes in record in ascii hex
aaaa == address to load data record at in memory
tt == type of record; 00=data; 01=end of file
ddd...d == data bytes of record in ascii hex (two chars per byte)
cc == checksum of record
checksum is two's complement of eight bit sum of all data from 'nn'
to end of data '...d'
end of file record (00) has zero number of bytes
So the above record would be decoded as :
:090160001204283119740280E236
start of record :
length of record 09
address to store record at 0160
record type 00
actual data of record 1204283119740280E2
checksum of record 36
Wednesday, July 16, 2008
Find error number in C
Refer : http://www.xinotes.net/notes/note/1793/ for getsockopt case
/usr/include/asm/error.h
#ifndef _I386_ERRNO_H
#define _I386_ERRNO_H
#define EPERM 1 /* Operation not permitted */
#define ENOENT 2 /* No such file or directory */
#define ESRCH 3 /* No such process */
#define EINTR 4 /* Interrupted system call */
#define EIO 5 /* I/O error */
#define ENXIO 6 /* No such device or address */
#define E2BIG 7 /* Arg list too long */
#define ENOEXEC 8 /* Exec format error */
#define EBADF 9 /* Bad file number */
#define ECHILD 10 /* No child processes */
#define EAGAIN 11 /* Try again */
#define ENOMEM 12 /* Out of memory */
#define EACCES 13 /* Permission denied */
#define EFAULT 14 /* Bad address */
#define ENOTBLK 15 /* Block device required */
#define EBUSY 16 /* Device or resource busy */
#define EEXIST 17 /* File exists */
#define EXDEV 18 /* Cross-device link */
#define ENODEV 19 /* No such device */
#define ENOTDIR 20 /* Not a directory */
#define EISDIR 21 /* Is a directory */
#define EINVAL 22 /* Invalid argument */
#define ENFILE 23 /* File table overflow */
#define EMFILE 24 /* Too many open files */
#define ENOTTY 25 /* Not a typewriter */
#define ETXTBSY 26 /* Text file busy */
#define EFBIG 27 /* File too large */
#define ENOSPC 28 /* No space left on device */
#define ESPIPE 29 /* Illegal seek */
#define EROFS 30 /* Read-only file system */
#define EMLINK 31 /* Too many links */
#define EPIPE 32 /* Broken pipe */
#define EDOM 33 /* Math argument out of domain of func */
#define ERANGE 34 /* Math result not representable */
#define EDEADLK 35 /* Resource deadlock would occur */
#define ENAMETOOLONG 36 /* File name too long */
#define ENOLCK 37 /* No record locks available */
#define ENOSYS 38 /* Function not implemented */
#define ENOTEMPTY 39 /* Directory not empty */
#define ELOOP 40 /* Too many symbolic links encountered */
#define EWOULDBLOCK EAGAIN /* Operation would block */
#define ENOMSG 42 /* No message of desired type */
#define EIDRM 43 /* Identifier removed */
#define ECHRNG 44 /* Channel number out of range */
#define EL2NSYNC 45 /* Level 2 not synchronized */
#define EL3HLT 46 /* Level 3 halted */
#define EL3RST 47 /* Level 3 reset */
#define ELNRNG 48 /* Link number out of range */
#define EUNATCH 49 /* Protocol driver not attached */
#define ENOCSI 50 /* No CSI structure available */
#define EL2HLT 51 /* Level 2 halted */
#define EBADE 52 /* Invalid exchange */
#define EBADR 53 /* Invalid request descriptor */
#define EXFULL 54 /* Exchange full */
#define ENOANO 55 /* No anode */
#define EBADRQC 56 /* Invalid request code */
#define EBADSLT 57 /* Invalid slot */
#define EDEADLOCK EDEADLK
#define EBFONT 59 /* Bad font file format */
#define ENOSTR 60 /* Device not a stream */
#define ENODATA 61 /* No data available */
#define ETIME 62 /* Timer expired */
#define ENOSR 63 /* Out of streams resources */
#define ENONET 64 /* Machine is not on the network */
#define ENOPKG 65 /* Package not installed */
#define EREMOTE 66 /* Object is remote */
#define ENOLINK 67 /* Link has been severed */
#define EADV 68 /* Advertise error */
#define ESRMNT 69 /* Srmount error */
#define ECOMM 70 /* Communication error on send */
#define EPROTO 71 /* Protocol error */
#define EMULTIHOP 72 /* Multihop attempted */
#define EDOTDOT 73 /* RFS specific error */
#define EBADMSG 74 /* Not a data message */
#define EOVERFLOW 75 /* Value too large for defined data type */
#define ENOTUNIQ 76 /* Name not unique on network */
#define EBADFD 77 /* File descriptor in bad state */
#define EREMCHG 78 /* Remote address changed */
#define ELIBACC 79 /* Can not access a needed shared library */
#define ELIBBAD 80 /* Accessing a corrupted shared library */
#define ELIBSCN 81 /* .lib section in a.out corrupted */
#define ELIBMAX 82 /* Attempting to link in too many shared libraries */
#define ELIBEXEC 83 /* Cannot exec a shared library directly */
#define EILSEQ 84 /* Illegal byte sequence */
#define ERESTART 85 /* Interrupted system call should be restarted */
#define ESTRPIPE 86 /* Streams pipe error */
#define EUSERS 87 /* Too many users */
#define ENOTSOCK 88 /* Socket operation on non-socket */
#define EDESTADDRREQ 89 /* Destination address required */
#define EMSGSIZE 90 /* Message too long */
#define EPROTOTYPE 91 /* Protocol wrong type for socket */
#define ENOPROTOOPT 92 /* Protocol not available */
#define EPROTONOSUPPORT 93 /* Protocol not supported */
#define ESOCKTNOSUPPORT 94 /* Socket type not supported */
#define EOPNOTSUPP 95 /* Operation not supported on transport endpoint */
#define EPFNOSUPPORT 96 /* Protocol family not supported */
#define EAFNOSUPPORT 97 /* Address family not supported by protocol */
#define EADDRINUSE 98 /* Address already in use */
#define EADDRNOTAVAIL 99 /* Cannot assign requested address */
#define ENETDOWN 100 /* Network is down */
#define ENETUNREACH 101 /* Network is unreachable */
#define ENETRESET 102 /* Network dropped connection because of reset */
#define ECONNABORTED 103 /* Software caused connection abort */
#define ECONNRESET 104 /* Connection reset by peer */
#define ENOBUFS 105 /* No buffer space available */
#define EISCONN 106 /* Transport endpoint is already connected */
#define ENOTCONN 107 /* Transport endpoint is not connected */
#define ESHUTDOWN 108 /* Cannot send after transport endpoint shutdown */
#define ETOOMANYREFS 109 /* Too many references: cannot splice */
#define ETIMEDOUT 110 /* Connection timed out */
#define ECONNREFUSED 111 /* Connection refused */
#define EHOSTDOWN 112 /* Host is down */
#define EHOSTUNREACH 113 /* No route to host */
#define EALREADY 114 /* Operation already in progress */
#define EINPROGRESS 115 /* Operation now in progress */
#define ESTALE 116 /* Stale NFS file handle */
#define EUCLEAN 117 /* Structure needs cleaning */
#define ENOTNAM 118 /* Not a XENIX named type file */
#define ENAVAIL 119 /* No XENIX semaphores available */
#define EISNAM 120 /* Is a named type file */
#define EREMOTEIO 121 /* Remote I/O error */
#define EDQUOT 122 /* Quota exceeded */
#define ENOMEDIUM 123 /* No medium found */
#define EMEDIUMTYPE 124 /* Wrong medium type */
#endif
/usr/include/asm/error.h
#ifndef _I386_ERRNO_H
#define _I386_ERRNO_H
#define EPERM 1 /* Operation not permitted */
#define ENOENT 2 /* No such file or directory */
#define ESRCH 3 /* No such process */
#define EINTR 4 /* Interrupted system call */
#define EIO 5 /* I/O error */
#define ENXIO 6 /* No such device or address */
#define E2BIG 7 /* Arg list too long */
#define ENOEXEC 8 /* Exec format error */
#define EBADF 9 /* Bad file number */
#define ECHILD 10 /* No child processes */
#define EAGAIN 11 /* Try again */
#define ENOMEM 12 /* Out of memory */
#define EACCES 13 /* Permission denied */
#define EFAULT 14 /* Bad address */
#define ENOTBLK 15 /* Block device required */
#define EBUSY 16 /* Device or resource busy */
#define EEXIST 17 /* File exists */
#define EXDEV 18 /* Cross-device link */
#define ENODEV 19 /* No such device */
#define ENOTDIR 20 /* Not a directory */
#define EISDIR 21 /* Is a directory */
#define EINVAL 22 /* Invalid argument */
#define ENFILE 23 /* File table overflow */
#define EMFILE 24 /* Too many open files */
#define ENOTTY 25 /* Not a typewriter */
#define ETXTBSY 26 /* Text file busy */
#define EFBIG 27 /* File too large */
#define ENOSPC 28 /* No space left on device */
#define ESPIPE 29 /* Illegal seek */
#define EROFS 30 /* Read-only file system */
#define EMLINK 31 /* Too many links */
#define EPIPE 32 /* Broken pipe */
#define EDOM 33 /* Math argument out of domain of func */
#define ERANGE 34 /* Math result not representable */
#define EDEADLK 35 /* Resource deadlock would occur */
#define ENAMETOOLONG 36 /* File name too long */
#define ENOLCK 37 /* No record locks available */
#define ENOSYS 38 /* Function not implemented */
#define ENOTEMPTY 39 /* Directory not empty */
#define ELOOP 40 /* Too many symbolic links encountered */
#define EWOULDBLOCK EAGAIN /* Operation would block */
#define ENOMSG 42 /* No message of desired type */
#define EIDRM 43 /* Identifier removed */
#define ECHRNG 44 /* Channel number out of range */
#define EL2NSYNC 45 /* Level 2 not synchronized */
#define EL3HLT 46 /* Level 3 halted */
#define EL3RST 47 /* Level 3 reset */
#define ELNRNG 48 /* Link number out of range */
#define EUNATCH 49 /* Protocol driver not attached */
#define ENOCSI 50 /* No CSI structure available */
#define EL2HLT 51 /* Level 2 halted */
#define EBADE 52 /* Invalid exchange */
#define EBADR 53 /* Invalid request descriptor */
#define EXFULL 54 /* Exchange full */
#define ENOANO 55 /* No anode */
#define EBADRQC 56 /* Invalid request code */
#define EBADSLT 57 /* Invalid slot */
#define EDEADLOCK EDEADLK
#define EBFONT 59 /* Bad font file format */
#define ENOSTR 60 /* Device not a stream */
#define ENODATA 61 /* No data available */
#define ETIME 62 /* Timer expired */
#define ENOSR 63 /* Out of streams resources */
#define ENONET 64 /* Machine is not on the network */
#define ENOPKG 65 /* Package not installed */
#define EREMOTE 66 /* Object is remote */
#define ENOLINK 67 /* Link has been severed */
#define EADV 68 /* Advertise error */
#define ESRMNT 69 /* Srmount error */
#define ECOMM 70 /* Communication error on send */
#define EPROTO 71 /* Protocol error */
#define EMULTIHOP 72 /* Multihop attempted */
#define EDOTDOT 73 /* RFS specific error */
#define EBADMSG 74 /* Not a data message */
#define EOVERFLOW 75 /* Value too large for defined data type */
#define ENOTUNIQ 76 /* Name not unique on network */
#define EBADFD 77 /* File descriptor in bad state */
#define EREMCHG 78 /* Remote address changed */
#define ELIBACC 79 /* Can not access a needed shared library */
#define ELIBBAD 80 /* Accessing a corrupted shared library */
#define ELIBSCN 81 /* .lib section in a.out corrupted */
#define ELIBMAX 82 /* Attempting to link in too many shared libraries */
#define ELIBEXEC 83 /* Cannot exec a shared library directly */
#define EILSEQ 84 /* Illegal byte sequence */
#define ERESTART 85 /* Interrupted system call should be restarted */
#define ESTRPIPE 86 /* Streams pipe error */
#define EUSERS 87 /* Too many users */
#define ENOTSOCK 88 /* Socket operation on non-socket */
#define EDESTADDRREQ 89 /* Destination address required */
#define EMSGSIZE 90 /* Message too long */
#define EPROTOTYPE 91 /* Protocol wrong type for socket */
#define ENOPROTOOPT 92 /* Protocol not available */
#define EPROTONOSUPPORT 93 /* Protocol not supported */
#define ESOCKTNOSUPPORT 94 /* Socket type not supported */
#define EOPNOTSUPP 95 /* Operation not supported on transport endpoint */
#define EPFNOSUPPORT 96 /* Protocol family not supported */
#define EAFNOSUPPORT 97 /* Address family not supported by protocol */
#define EADDRINUSE 98 /* Address already in use */
#define EADDRNOTAVAIL 99 /* Cannot assign requested address */
#define ENETDOWN 100 /* Network is down */
#define ENETUNREACH 101 /* Network is unreachable */
#define ENETRESET 102 /* Network dropped connection because of reset */
#define ECONNABORTED 103 /* Software caused connection abort */
#define ECONNRESET 104 /* Connection reset by peer */
#define ENOBUFS 105 /* No buffer space available */
#define EISCONN 106 /* Transport endpoint is already connected */
#define ENOTCONN 107 /* Transport endpoint is not connected */
#define ESHUTDOWN 108 /* Cannot send after transport endpoint shutdown */
#define ETOOMANYREFS 109 /* Too many references: cannot splice */
#define ETIMEDOUT 110 /* Connection timed out */
#define ECONNREFUSED 111 /* Connection refused */
#define EHOSTDOWN 112 /* Host is down */
#define EHOSTUNREACH 113 /* No route to host */
#define EALREADY 114 /* Operation already in progress */
#define EINPROGRESS 115 /* Operation now in progress */
#define ESTALE 116 /* Stale NFS file handle */
#define EUCLEAN 117 /* Structure needs cleaning */
#define ENOTNAM 118 /* Not a XENIX named type file */
#define ENAVAIL 119 /* No XENIX semaphores available */
#define EISNAM 120 /* Is a named type file */
#define EREMOTEIO 121 /* Remote I/O error */
#define EDQUOT 122 /* Quota exceeded */
#define ENOMEDIUM 123 /* No medium found */
#define EMEDIUMTYPE 124 /* Wrong medium type */
#endif
Monday, June 9, 2008
Telnet command with port number.
Telnet command will helps to peep in to the remote server's application port. And we can have response from the remote server if you sent appropriate packets or data.
Syntax: telnet [IP address] [Application port num]
eg:
#telnet 192.168.1.2 8000
[ type here to send the appropriate data which remote server can recognize ]
Use Crtl + ] to get command mode prompt.
telnet> ? Enter
Gives some commad
telnet>close -->to exit
If you want to send Http response from command ..
Use ethereal to capture the data to be sent.
[Hint Right click the request and select TCP Streams ,You will get the Http response packet , Just Copy and paste it after telnet.]
Procedure
---------
Below packet is the capture of the HTTP get method ,since
assuming httpd is running in the server at port 8080.
#telnet 192.168.1.2 8080
If application is HTTP server below code capture will help full.
---This is what HTTP GET method request packet looks----
GET /index.html HTTP/1.1
Accept: */*
Accept-Language: en-us
Accept-Encoding: gzip, deflate
User-Agent: Mozilla/4.0 (compatible; MSIE 5.01; Windows NT 5.0)
Host: 192.168.1.1:8080
Connection: Keep-Alive
Cache-Control: no-cache
------------------------Ends-----------------
---This is what HTTP POST method request packet looks----
POST /line_sip.htm?l=1 HTTP/1.1
Content-Length: 312
Connection: Keep-Alive
user_moh1=&user_alert_info1=&user_pic1=&user_dp_str1= &user_dp_exp1_0=off&user_dper_expiry1=3600&user_auto_connect1=off &user_descr_contact1=on&user_sipusername_and_local_name1=off& Settings=Save
------------------------Ends-----------------
user_moh1,user_alert_info1 .. are variables getting update values by POST request.
Syntax: telnet [IP address] [Application port num]
eg:
#telnet 192.168.1.2 8000
[ type here to send the appropriate data which remote server can recognize ]
Use Crtl + ] to get command mode prompt.
telnet> ? Enter
Gives some commad
telnet>close -->to exit
If you want to send Http response from command ..
Use ethereal to capture the data to be sent.
[Hint Right click the request and select TCP Streams ,You will get the Http response packet , Just Copy and paste it after telnet.]
Procedure
---------
Below packet is the capture of the HTTP get method ,since
assuming httpd is running in the server at port 8080.
#telnet 192.168.1.2 8080
If application is HTTP server below code capture will help full.
---This is what HTTP GET method request packet looks----
GET /index.html HTTP/1.1
Accept: */*
Accept-Language: en-us
Accept-Encoding: gzip, deflate
User-Agent: Mozilla/4.0 (compatible; MSIE 5.01; Windows NT 5.0)
Host: 192.168.1.1:8080
Connection: Keep-Alive
Cache-Control: no-cache
------------------------Ends-----------------
---This is what HTTP POST method request packet looks----
POST /line_sip.htm?l=1 HTTP/1.1
Content-Length: 312
Connection: Keep-Alive
user_moh1=&user_alert_info1=&user_pic1=&user_dp_str1= &user_dp_exp1_0=off&user_dper_expiry1=3600&user_auto_connect1=off &user_descr_contact1=on&user_sipusername_and_local_name1=off& Settings=Save
------------------------Ends-----------------
user_moh1,user_alert_info1 .. are variables getting update values by POST request.
Telephone Recorder Circuit
Friday, June 6, 2008
Test Operator Usage
Thursday, April 3, 2008
Linux Commad ctags with find
catgs:
ctags -w `find $(SUBDIRS) include common \( -name CVS -prune \) -o \( -name '*.[ch]' -print \)`
find [options]
-name '*.[ch]' => This option restricts *.c and *.h files only
-prune -o -print => use to display and cut out subdirectories
This will search only .c and .h files with argument as search pattern"
alias f "find . -name '*.[c,h]' -print | xargs grep -n --color $1 "
eg: f pattern
ctags -w `find $(SUBDIRS) include common \( -name CVS -prune \) -o \( -name '*.[ch]' -print \)`
find [options]
-name '*.[ch]' => This option restricts *.c and *.h files only
-prune -o -print => use to display and cut out subdirectories
This will search only .c and .h files with argument as search pattern"
alias f "find . -name '*.[c,h]' -print | xargs grep -n --color $1 "
eg: f pattern
Saturday, March 22, 2008
VoIP-H.323
The Media Gateway (MGW) table holds information about each media gateway managed by the Call Agent. The media gateway can be uniquely addressed by domain name, an IP address, or the TSAP address.
Megaco/H.248 protocol: history

The Media Gateway table has two associated commands: RGW and TGW. The RGW command provisions a gateway as only a Residential GateWay, with the type token automatically set to RGW. The TGW command provisions a gateway as a Trunking GateWay only, with the type token automatically set to TGW. Both of these commands provision the Media Gateway table, but a service provider can use these commands to provide user security to certain individuals based on their roles.
H.323
------
H.225 also for RAS( Registration ,admission,Status)
H.225/Q.931 can be defined as signaling (eg.call setup)
H.245 can be defined as control signaling (eg .Terminal cabability setup )
H.320 can be defined as multimedia service over ISDN
H.321 or H.310 can be defined as multimedia service over B-ISDN
H.322 can be defined as multimedia service over QoS Garenteed networks
H.323 can be defined as multimedia service over packet networks
H.323 can be defined as multimedia service over SCN

Megaco/H.248 protocol: history
The Media Gateway table has two associated commands: RGW and TGW. The RGW command provisions a gateway as only a Residential GateWay, with the type token automatically set to RGW. The TGW command provisions a gateway as a Trunking GateWay only, with the type token automatically set to TGW. Both of these commands provision the Media Gateway table, but a service provider can use these commands to provide user security to certain individuals based on their roles.
H.323
------
H.225 also for RAS( Registration ,admission,Status)
H.225/Q.931 can be defined as signaling (eg.call setup)
H.245 can be defined as control signaling (eg .Terminal cabability setup )
H.320 can be defined as multimedia service over ISDN
H.321 or H.310 can be defined as multimedia service over B-ISDN
H.322 can be defined as multimedia service over QoS Garenteed networks
H.323 can be defined as multimedia service over packet networks
H.323 can be defined as multimedia service over SCN

Sunday, March 9, 2008
Badminton Central Guide to choosing Badminton Equipment
I have wanted to write a guide on what equipment badminton should focus on. By nature, badminton requires the player to have a few pieces of equipment to engage in the sports, items such as a racket and a shuttlecock are a must for a badminton player. However, often I see many player places the wrong focus on how they spend their money on equipment. A smart badminton player will spend money effectively to maximize their badminton playing experience.
However, before I start, I would like to point out that no badminton equipment can replace proper badminton skills. If you think you can spend US$200 on a racket and you can instantly play better, you are 105% wrong. A good set of equipment can only bring out the potential of a player with good skills. No equipment can fix your bad skills. Instead, I recommend you spend your money on some good coaching lessons. It will make much more difference in your badminton game than a shiny new racket.
I chose to list the equipment in decreasing order of important, in other words, I reckon that the badminton shoes is the most important equipment that a badminton player has, while their clothing is the least important.
Shoes:
======
Contrary to popular belief, a good set of badminton shoes are the most important piece of equipment a badminton player can have. Badminton players move around the badminton court at an amazing pace, dashes and changes directions on every stroke of the rally, twisting and turning and lunging. All these movements are supported and made possible by the biggest unsung hero, the badminton shoes.
Badminton shoes are designed for badminton movements; a thin but well supported sole with good lateral support keep the player's feet close to the ground, this allows for fast and ankle bending directional changes with lower chance of injury; light weight for faster feet movement; surface hugging gummy soles to grip the indoor surface without slipping.
The amount of torture we subject our lower body to can clearly be seen in people's badminton shoes. A good pair of badminton shoes used by a decent player sometimes last only 3-6 months. At the end of its short life, you will find soles that are worn to the inner support, insoles that are worn through, strong upper leather that gave up and split due to the immense force exerted at it.
Pick your badminton shoes with care, make sure you choose the pair that fits the shape of your feet and thus the most comfortable.
And whatever you do, do not wear running shoes or any other thick soled shoes. They keep your feet too high up in the ground and all it will take is one deep lunge to have your ankle sprained. From then on, you will be in excruciating pain for days and the injury will affect your badminton game for years to come.
Good badminton shoes brands include
Asics,
Hi-Tec,
Mizuno, and
Yonex. Other smaller brands are starting to catching up as well.
String:
=======
The number two unsung hero of the badminton equipment is the badminton string. Afterall, it is the string that is in contact with the shuttle on every stroke. How the string interacts with the shuttle is crucial to the feel of each stroke. Depending on your skill level and the style of your game, you should pick a string and tension that is suitable for your game. String manufacturers usually have ratings of different string characteristics at the back of the string package. Pick the items that are most important for your game.
String tension affects the playability of the string as much as the string itself. The general rule of thumb is that the harder you can hit, the tighter your tension can be. A higher tension rewards a hard hitting while robs power from a light hitter. On the opposite end, a lower tensioned string helps light hitter with a better timed trampoline effect.
There is always the temptation to go higher in tension, but this is a case of bigger is not always better. Higher tension does not give you more power as mentioned above, beginners should always start with lower tension of around 20lbs, adjust it to 22-23lbs when you progress to intermediate and only go up to 25+lbs if you are gain more power in your technique. Using the inappropriate high tension will make the racket unresponsive, decreased power, and will easily cause injury.
Good brands for badminton strings are: Ashaway, Gosen, Mizuno, Yonex.
Recommended tensions:
beginners: 19-20lbs .(i.e. 2.2 lbs == 1 kg)
intermediate players: 21-24lbs.
advance players: 25+ lbs.
Grip:
=====
The number three unsung hero of badminton equipment is the badminton grip. Similar to the string being the interface to the shuttle, the grip is the interface to the badminton players finger and hand. The game of badminton comprises of many very delicate movements and fine control from the players€™s finger and wrist. A proper grip ensure that there is proper actuation and feedback to and from the racket.
Three major factors affects the characteristics of the grip: type, size and tackiness.
Badminton grips falls into two different type, towel and synthetic. The choice of which are personal preference. Towel grip are softer, provides good sweat absorption, but at the same time, is more prone to germ accumulation and needs to be changed often. Synthetic grips are less messy and less prone to accumulation of germs, however, they are not as good as towel grips when it comes to sweat absorption.
The proper grip size to use depends greatly on the player. Obviously a player with larger hands will prefer a larger grip and vice versa. It is often tempting to use a large grip, a large grip gives the false feeling that the racket is lighter and more maneuverable. However, one must again understand that badminton is comprised of subtle, agile and delicate movements in the fingers and wrist, a smaller (but not too small) grip will allow for higher agility and maneuverability of the racket.
Racquet:
=======
Often times badminton players give the racket the most emphasis. If you go into http://www.badmintoncentral.com and you will find that most of the discussion centers on the racket. While the racket is certainly an important equipment, the importance of which is often overrated, and that is the reason why I put the Racket almost next to last in our recommendation list.
The badminton racket is the middleman when transferring force from the player to the shuttle, sitting right between the grip and the string. A badminton racket can be categorized by a few characteristics: shape, stiffness, weight, balance.
Virtually all badminton rackets are made of carbon fiber or graphite. Some manufacturers choose to put in extra ingredients into the racket material like titanium or recently nanocarbon. I want to emphasis that they are only addictives, the 99% of the racket is still graphite and the extra benefits of the additives are very marginal.
Badminton rackets comes in two major head shapes:
1) Isometric/square and
2) Oval.
Oval is the traditional racket shape, it is a slightly bottom heavy oval, almost the shape of an egg. Oval rackets in general have a small but more concentrated sweet spot. Oval fans like the concentration of power around the sweetspot of the racket.
The shape is the Isometric or square which became more popular after the early 1990̢۪s. The Isometric head has a wider and more squared top half of the racket head. The advantage of the isometric is an enlarged sweetspot which give off-centered hit a better response.
The effective stiffness of a badminton racket is similar to the effective of string tension. So I will refer you back to that section. A stiffer racket has the similar effect as a higher tensioned string, while a flexible racket is similar to the lower tension string.
Rackets comes in different weights. Normally the racket alone weighs between 80-95g. Different manufacturers have different rating system, the most popular of which is Yonex̢۪s U system,
Weight Size | Weight (g) | Main Use |
---|---|---|
1U | 95-99.9 | Training Racquets |
2U | 90-94.9 | Attacking Singles |
3U | 85-89.9 | Singles/Attacking Doubles |
4U | 80-84.9 | Doubles/Defensive Singles |
5U | 75-79.9 | Defensive Doubles |
a racket's weight determines how fast one can swing a racket, the lighter a racket, the faster one can swing it with the same force. In general, a lighter racket is more maneuverable than a heavy one. However, before everybody goes out and buy the lightest racket, I also want to point out that lighter isn̢۪t always better. A light racket is less stable than a heavier racket, more force is necessary to keep its path, furthermore, a heavier racket has a larger momentum and thus more effective in transferring its speed and power to the shuttle.
The final racket characteristic is the balance of the racket. Head balanced racket is becoming more popular recently. Head balanced rackets have more mass near the head of the racket. A head balanced racket is more stable and have higher angular momentum when swung. On the contrary, a even balanced racket is more maneuverable.
As you can see, none of the different properties of the above characteristics are strictly better than other. Some players prefers slightly heavier rackets, some prefer slightly lighter, some like even balanced, some like head balanced. What I want to point out is that ultimately, it is a person̢۪s skill level and style that determines what racket is suitable, go try them out if you can to see what fits.
Clothing
======
Badminton clothing is quite simple to choose. Aside from one personal fashion preference, badminton clothing is better kept light and unrestrictive. Shirts needs to be slightly loose and comfortable to allow for arm and body movements. Badminton is a game of sweat, very often we see a badminton player walks out of the court like he just came out of a shower. As it is always uncomfortable to have sweat stuck to one̢۪s skin, sweat absorption and dissipation is thus important. There are many fancy sweat-wicking clothing out there, but from my personal experience, a good cotton t-shirt works well in most situation. If one gets really wet during a game, they are cheap enough so you can have a few spares ones to change into.
Shorts are the preference for most badminton players. Again, choose something that is light and not restrictive to movement, jumps and lunges are done often in badminton.
Again, clothing is mostly personal fashion preference, as long as the clothing allows for the extreme movements in badminton, it is usually quite ok.
I have mentioned it once and I will do it again here, while equipment is essential in badminton, the most important factor in badminton is still one̢۪s badminton skills. Equipment will only have marginal effect on one̢۪s game.
Source: Badminton Central
Guide for Shuttle rules:
http://www.annandale.k12.mn.us/AHS/faculty/pamfliegel/webpage/Badminton%20Study%20Guide1.htm
Compare Yonex Strings
Now let's discuss the string. There are many different brand of string. But Yonex is more widely used. The following is mainly translated from a post by someone who have strung more than 20,000 racquets for professional teams in China. Unfortunately, the original post has been copied too many times. I cannot find his name or contact. Anyway, I strongly appreciate his detailed and professional comment on almost all Yonex strings.I focus on those available on US market. For those I used, I may add some of my own comment. I may also skip some details I think less important.
=====================================================================
First of all. I don't recommend Ti65 and Ti68 string. Except for a more crispy sound, there is nothing good about it. More importantly, the Ti skin on the surface of the string change the consistency of elasticiy of the string. That makes it fragile. The Ti skin also covers up the texture of the string. That reduced the control. Due to the sound, it makes you feel more powerful when you smash. But that is mostly hallucination only.(Normally this is used by professional players. They will restring after the game. If you can afford to do that. Ti string may still be a good choice for you.)
BG65 is most widely used. Mainly because it is the one used by National team for training purpose. But the BG65 used by national team is not the one in seperate bag we found on the market. It is the big reel version. (I guess the 200m Reel)The bag version is softer and smoother. Overall, it is very durable. It provides a good balance between quality and economy
I prefer BG66. It is a very thin string. Only 0.66mm.Extrodinary recillience. Good for beginer or players with relative less power. Cons: It is too thin. So it is easy to break. Also the control is not quite good.
In my opinion, BG70 is not a successful product. Yonex want to make it omnipotent: good recillience, good control and durable. Finally, nothing is very good.
BG80 is a .68mm string. Very good recillience. It is excellent for offensive plan. You can combine it with offensive racquets like NanoSpeed 9000, AT700, Ti10, MP100, etc. Strongly recommended for offensive player. One unqiue feature of BG80 is that you can get the feeling of a string setup at higher tension. (I use BG80 with my Ti10 at 24lb. It is a pretty powerful combination.)
BG85 is relative soft. It has a higher thread count. Its control is excellent, with the recillience second to only BG66. It is good for defensive player.
BGN95. This is not reviewed by the original post. But I used it with my NS9000 at 24lb. Recillience is not as good as BG80. But it is still pretty good overall. Good control and durability. It break only once when my racquet collide with partner's.
Model | Recillience | Feel | Shock Absorption | Control | Durability |
BG-65 | B | Medium | A | A | A |
BG-65Ti | A | Stiff | B | A | A |
BG-66 | A+ | Stiff | A+ | B | B |
BG-68Ti | A+ | Verfy Stiff | A+ | A+ | B |
BG-70Pro | A | Medium | B | A | A |
BG-80 | A+ | Stiff | A | A | A |
BG-85 | A+ | Very Stiff | A | A | C |
BG-95 | A | Stiff | A+ | A+ | A |
Shuttle cork:
Following are the Key Benefits of YONEX Mavis Series of Nylon Shuttlecock:
•Economical and durable Shuttlecock performance.
•Best Shuttle Flight and Characteristics (Close to Feather Shuttle).
•Better Flight pattern (Better then other Nylon shuttles and close to feather shuttlecocks).
•YONEX Mavis shuttlecock recovers in .02 secs (This fast shuttle recovery is better then other brand (.008 sec faster) , while its only .005 sec slower then feather shuttlecock)
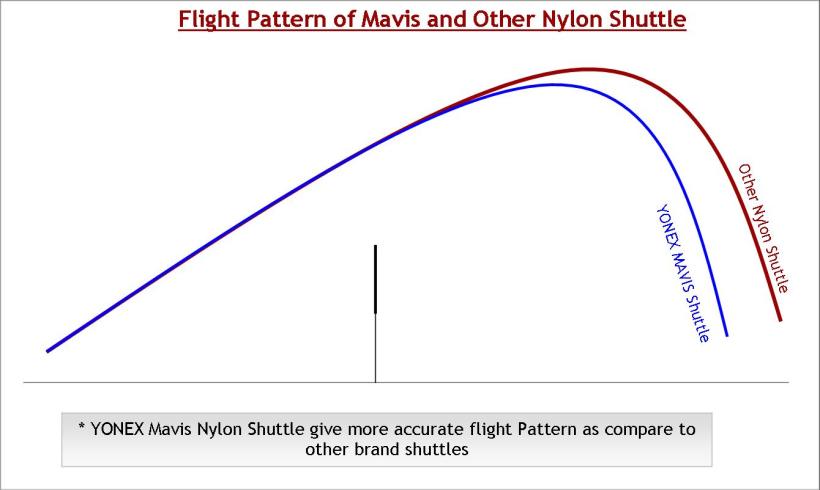
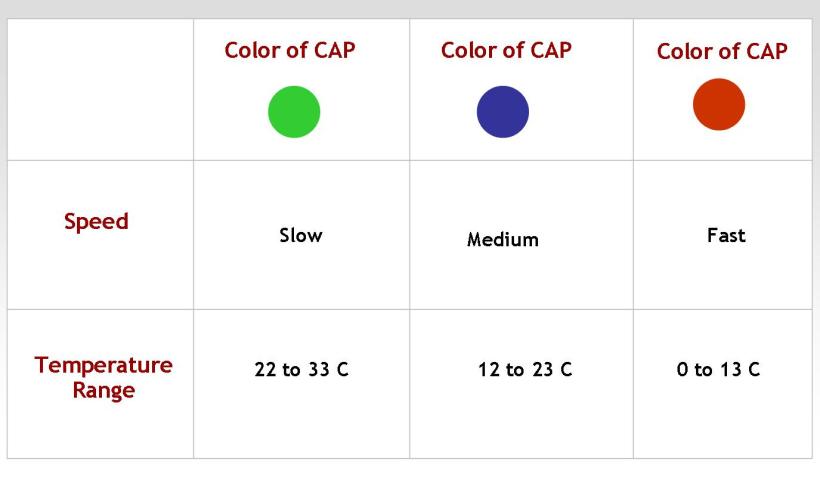
Thursday, March 6, 2008
Pthread Creation and Termination
Example: Pthread Creation and Termination
gcc example.c -pthread
#include pthread.h
#include stdio.h
#define NUM_THREADS 5
void *PrintHello(void *threadid)
{
int tid;
tid = (int)threadid;
printf("Hello World! It's me, thread #%d!\n", tid);
pthread_exit(NULL);
}
int main (int argc, char *argv[])
{
pthread_t threads[NUM_THREADS];
int rc, t;
for(t=0; t<= NUM_THREADS; t++){
printf("In main: creating thread %d\n", t);
rc = pthread_create(&threads[t], NULL, PrintHello, (void *)t);
if (rc){
printf("ERROR; return code from pthread_create() is %d\n", rc);
exit(-1);
}
}
pthread_exit(NULL);
}
gcc example.c -pthread
#include pthread.h
#include stdio.h
#define NUM_THREADS 5
void *PrintHello(void *threadid)
{
int tid;
tid = (int)threadid;
printf("Hello World! It's me, thread #%d!\n", tid);
pthread_exit(NULL);
}
int main (int argc, char *argv[])
{
pthread_t threads[NUM_THREADS];
int rc, t;
for(t=0; t<= NUM_THREADS; t++){
printf("In main: creating thread %d\n", t);
rc = pthread_create(&threads[t], NULL, PrintHello, (void *)t);
if (rc){
printf("ERROR; return code from pthread_create() is %d\n", rc);
exit(-1);
}
}
pthread_exit(NULL);
}
Thursday, February 21, 2008
Audio Companding technique : G.711- A-Law
A-Law and u-law is meant for 8-bit raw linear data only.
Supported by G.711 format.
To Know more
http://en.wikipedia.org/wiki/G.711
Sampling frequency 8 kHz
Supported by G.711 format.
To Know more
http://en.wikipedia.org/wiki/G.711
Sampling frequency 8 kHz
Enabling interupt in LPC2212
/* This hold entire Application notes */
http://www.nxp.com/pip/LPC2212_2214_4.html
/* Manual : http://www.keil.com/dd/chip/3649.htm */
TN06002_LPC2000_EINT.pdf contains Below Code
int main (void)
{
IODIR1 = 0x00010000; // P1.16 defined as GPO
PINSEL1 |= 0x00000001; // P0.16 as EINT0 interrupt pin
VPBDIV = 0;
EXTMODE = 0x01; // EINT0 is (falling) edge-sensitive
// VPBDIV = 0x01; // additional step see errata
// VPB clock = CPU clock
VICVectAddr0 = (unsigned int) &EINT0_Isr;
EXTINT = 0x01; // Clear the peripheral interrupt flag
VICVectCntl0 = 0x2E; // Channel0 on Source# 14 == 0xE... enabled
/* Channel0 - high priority
Channel15 - low priority */
VICIntEnable = 0x4000; // 14th bit is EINT0
while (1) ;
}
void EINT0_Isr(void) __irq // for external interrupt 0
{
VICIntEnClr = 0x00004000; // Disable EINT0 in the VIC
/*.............
Our code
....*/
EXTINT = 0x01; // Clear the peripheral interrupt flag
VICIntEnable = 0x00004000; // Enable EINT0 in the VIC I.e 14 bit ==VIC# 14
VICVectAddr = 0; // reset VIC
}
http://www.nxp.com/pip/LPC2212_2214_4.html
/* Manual : http://www.keil.com/dd/chip/3649.htm */
TN06002_LPC2000_EINT.pdf contains Below Code
int main (void)
{
IODIR1 = 0x00010000; // P1.16 defined as GPO
PINSEL1 |= 0x00000001; // P0.16 as EINT0 interrupt pin
VPBDIV = 0;
EXTMODE = 0x01; // EINT0 is (falling) edge-sensitive
// VPBDIV = 0x01; // additional step see errata
// VPB clock = CPU clock
VICVectAddr0 = (unsigned int) &EINT0_Isr;
EXTINT = 0x01; // Clear the peripheral interrupt flag
VICVectCntl0 = 0x2E; // Channel0 on Source# 14 == 0xE... enabled
/* Channel0 - high priority
Channel15 - low priority */
VICIntEnable = 0x4000; // 14th bit is EINT0
while (1) ;
}
void EINT0_Isr(void) __irq // for external interrupt 0
{
VICIntEnClr = 0x00004000; // Disable EINT0 in the VIC
/*.............
Our code
....*/
EXTINT = 0x01; // Clear the peripheral interrupt flag
VICIntEnable = 0x00004000; // Enable EINT0 in the VIC I.e 14 bit ==VIC# 14
VICVectAddr = 0; // reset VIC
}
Friday, February 15, 2008
Linux Rare Comands
wget http_proxy=http://url:port --proxy-user=xxxxxx --proxy-password=xxxxxxx http://in2.php.net/distributions/php-5.2.6.tar.gz
To trace the process currently running ........
]# strace -p
To see the currently ports open
]# netstat -an
To trace the process currently running ........
]# strace -p
To see the currently ports open
]# netstat -an
Tuesday, January 22, 2008
Introduction to network functions in C
Introduction to network functions in C
http://shoe.bocks.com/net/
http://shoe.bocks.com/net/source/examples/chat.c
http://shoe.bocks.com/net/
http://shoe.bocks.com/net/source/examples/chat.c
Monday, January 21, 2008
kbhit() for Linux
/*
Well I would just use ncurses, but you could possibly use below? Chances are you problly don't need either unless you're making a crappy console game, or a password program.
*/
#include stdio.h>
#include termios.h>
#include unistd.h>
#include sys/types.h>
#include sys/time.h>
/* This id to hide the character we type and suspend any
prints after dir 1 , displayed ony when dir 0 */
void changemode ( int dir )
{
static struct termios oldt, newt;
if ( dir == 1 )
{
tcgetattr ( STDIN_FILENO, &oldt );
newt = oldt;
newt.c_lflag &= ~ ( ICANON | ECHO );
tcsetattr ( STDIN_FILENO, TCSANOW, &newt );
}
else
tcsetattr ( STDIN_FILENO, TCSANOW, &oldt );
}
int kbhit ( void )
{
struct timeval tv;
fd_set rdfs;
tv.tv_sec = 0;
tv.tv_usec = 0;
FD_ZERO ( &rdfs );
FD_SET ( STDIN_FILENO, &rdfs );
// select ( STDIN_FILENO + 1, &rdfs, NULL, NULL, &tv );
select ( STDIN_FILENO + 1, &rdfs, NULL, NULL, NULL );
printf(" After ");
return FD_ISSET ( STDIN_FILENO, &rdfs );
}
int main ( void )
{
int ch;
printf(" Press any Key to continue\n ");
changemode ( 1 );
while ( !kbhit() )
{
// putchar ( '.' );
}
ch = getchar();
printf ( "\nGot %c\n", ch );
changemode ( 0 );
return 0;
}
Well I would just use ncurses, but you could possibly use below? Chances are you problly don't need either unless you're making a crappy console game, or a password program.
*/
#include stdio.h>
#include termios.h>
#include unistd.h>
#include sys/types.h>
#include sys/time.h>
/* This id to hide the character we type and suspend any
prints after dir 1 , displayed ony when dir 0 */
void changemode ( int dir )
{
static struct termios oldt, newt;
if ( dir == 1 )
{
tcgetattr ( STDIN_FILENO, &oldt );
newt = oldt;
newt.c_lflag &= ~ ( ICANON | ECHO );
tcsetattr ( STDIN_FILENO, TCSANOW, &newt );
}
else
tcsetattr ( STDIN_FILENO, TCSANOW, &oldt );
}
int kbhit ( void )
{
struct timeval tv;
fd_set rdfs;
tv.tv_sec = 0;
tv.tv_usec = 0;
FD_ZERO ( &rdfs );
FD_SET ( STDIN_FILENO, &rdfs );
// select ( STDIN_FILENO + 1, &rdfs, NULL, NULL, &tv );
select ( STDIN_FILENO + 1, &rdfs, NULL, NULL, NULL );
printf(" After ");
return FD_ISSET ( STDIN_FILENO, &rdfs );
}
int main ( void )
{
int ch;
printf(" Press any Key to continue\n ");
changemode ( 1 );
while ( !kbhit() )
{
// putchar ( '.' );
}
ch = getchar();
printf ( "\nGot %c\n", ch );
changemode ( 0 );
return 0;
}
Monday, January 7, 2008
getche() equivalent in Linux
]# echo -n "Press any key to continue..." && read -n 1
or
]# read -sn1 -p " Press any key to continue "
Also :
]$ echo -n "Press any key to continue..." && /sbin/getkey
##test.sh
if /sbin/getkey -i -c 5 -m "Press Y within %d sec to continue " y;
then
echo You pressed y
else
echo You pressed Wrong
fi
echo
/sbin/getkey i && touch /var/run/confirm
if pressed key is 'i' return 1 else 0.
&& fail if 0 .
http://www.webservertalk.com/archive200-2005-7-1138374.html
or
]# read -sn1 -p " Press any key to continue "
Also :
]$ echo -n "Press any key to continue..." && /sbin/getkey
##test.sh
if /sbin/getkey -i -c 5 -m "Press Y within %d sec to continue " y;
then
echo You pressed y
else
echo You pressed Wrong
fi
echo
/sbin/getkey i && touch /var/run/confirm
if pressed key is 'i' return 1 else 0.
&& fail if 0 .
http://www.webservertalk.com/archive200-2005-7-1138374.html
Friday, January 4, 2008
Advanced Macro Tricks (Tokenizer )
Pasting Tokens
================
Each argument passed to a macro is a token, and sometimes it might be expedient to paste arguments together to form a new token. This could come in handy if you have a complicated structure and you'd like to debug your program by printing out different fields. Instead of writing out the whole structure each time, you might use a macro to pass in the field of the structure to print.
To paste tokens in a macro, use ## between the two things to paste together.
For instance
#define BUILD_FIELD(field) my_struct.inner_struct.union_a.##field
Now, when used with a particular field name, it will expand to something like
my_struct.inner_struct.union_a.field1
The tokens are literally pasted together.
String-izing Tokens
====================
Another potentially useful macro option is to turn a token into a string containing the literal text of the token. This might be useful for printing out the token. The syntax is simple--simply prefix the token with a pound sign (#).
#define PRINT_TOKEN(token) printf(#token " is %d", token)
For instance, PRINT_TOKEN(foo) would expand to
printf("foo" " is %d" foo)
(Note that in C, string literals next to each other are concatenated, so something like "token" " is " " this " will effectively become "token is this". This can be useful for formatting printf statements.)
For instance, you might use it to print the value of an expression as well as the expression itself (for debugging purposes).
PRINT_TOKEN(x + y);
http://www.cprogramming.com/tutorial/cpreprocessor.html
================
Each argument passed to a macro is a token, and sometimes it might be expedient to paste arguments together to form a new token. This could come in handy if you have a complicated structure and you'd like to debug your program by printing out different fields. Instead of writing out the whole structure each time, you might use a macro to pass in the field of the structure to print.
To paste tokens in a macro, use ## between the two things to paste together.
For instance
#define BUILD_FIELD(field) my_struct.inner_struct.union_a.##field
Now, when used with a particular field name, it will expand to something like
my_struct.inner_struct.union_a.field1
The tokens are literally pasted together.
String-izing Tokens
====================
Another potentially useful macro option is to turn a token into a string containing the literal text of the token. This might be useful for printing out the token. The syntax is simple--simply prefix the token with a pound sign (#).
#define PRINT_TOKEN(token) printf(#token " is %d", token)
For instance, PRINT_TOKEN(foo) would expand to
printf("foo" " is %d" foo)
(Note that in C, string literals next to each other are concatenated, so something like "token" " is " " this " will effectively become "token is this". This can be useful for formatting printf statements.)
For instance, you might use it to print the value of an expression as well as the expression itself (for debugging purposes).
PRINT_TOKEN(x + y);
http://www.cprogramming.com/tutorial/cpreprocessor.html
Thursday, January 3, 2008
Vb Script to automate the mail
Vb Script to automate the mail
------------------------------
SeeGood.vbs
-----------
Set WshShell = WScript.CreateObject("WScript.Shell")
WshShell.Run "Outlook"
WScript.Sleep 2500 ' Comment : Give Notepad some time to load
WshShell.SendKeys "^{n}" ' Ctrl+N
WScript.Sleep 1000
WshShell.SendKeys "test@mailid.com"
WshShell.SendKeys "{TAB}"
WshShell.SendKeys "{TAB}"
WshShell.SendKeys "{TAB}"
WScript.Sleep 100
WshShell.SendKeys " Hi,I would have checked out the code first! ....."
WshShell.SendKeys "{ENTER}"
WScript.Sleep 5000
WshShell.SendKeys "%{s}" 'Alt+s
'For
'SHIFT = +
'CTRL = ^
'ALT = %
------------------------------
SeeGood.vbs
-----------
Set WshShell = WScript.CreateObject("WScript.Shell")
WshShell.Run "Outlook"
WScript.Sleep 2500 ' Comment : Give Notepad some time to load
WshShell.SendKeys "^{n}" ' Ctrl+N
WScript.Sleep 1000
WshShell.SendKeys "test@mailid.com"
WshShell.SendKeys "{TAB}"
WshShell.SendKeys "{TAB}"
WshShell.SendKeys "{TAB}"
WScript.Sleep 100
WshShell.SendKeys " Hi,I would have checked out the code first! ....."
WshShell.SendKeys "{ENTER}"
WScript.Sleep 5000
WshShell.SendKeys "%{s}" 'Alt+s
'For
'SHIFT = +
'CTRL = ^
'ALT = %
Subscribe to:
Posts (Atom)